
Computer Science Projects
Give me 1 hour, data, and an IDE (a place to code), and watch the magic happen.
I learned how to code in high school, where I took programming classes (APCSA, APCSP), but frankly, I didn’t understand the power of programing in solving real-world problems.
Fast-forward to my first semester of college, where I went to my first Hackathon:
Rutgers Health Hackathon 2024. I had little programming experience, but in 48 hours, I learned: back-end development, generative artificial intelligence implementation, and front-end web development. I was the only Freshman on the team full of graduate students, medical students, MD’s and PHD’s, but I held my own, and we won 4th Place. Now, we’re working on developing our tool with Amazon Web Services and Robert Wood Johnson Hospital in New Jersey.
I tell everyone that I meet that the best way to get into programming and software engineering? Jump into a project. With a problem to solve and the world at your fingertips, programming will help you use technology to solve problems in fast, efficient, scalable ways.
Welcome to my Programming Portfolio, where technology meets real-world impact. From using machine learning in healthcare to predictive analytics in the automotive industry, my work focuses on using data to help people make smart decisions. I specialize in building efficient, scalable applications that solve practical problems across different fields.
Technology doesn’t have to be just about solving problems when they occur. I believe technology can be used as proactive measure to make our world more efficient, and expand the boundaries of the human race. Check out my work in technology below.
I love cars. So, I made some car-themed projects.
-
This program analyzes a BMW automotive dataset to predict stock price trends, optimize investment portfolios, test different trading strategies, and simulate real-market trading using machine learning and statistical models.
Click here to access this Project.
-
This program analyzes trends in car appearances in Hollywood over the decades. It creates interactive visualizations that show how different cars are featured in movies, and it uses machine learning to predict the genre of a movie based on the featured vehicle’s details, including: model, brand, and year. The program also recommends films based on selected vehicles and forecasts how popular certain cars might be in future movies.
Click here to access this Project.
-
This program recommends cars based on user preferences and criteria, providing insights like fuel efficiency and availability through an enhanced user interface.
Click here to access this Project.
-
This program classifies eco-friendly vehicles to assist consumers, analysts, and businesses in making sustainable, environmentally-conscious decisions.
Click here to access this Project.
-
This program classifies eco-friendly cars based on fuel type, consumption, and horsepower, helping consumers and businesses make environmentally conscious choices.
Click here to access this Project.
Forever a Celtics Girl.
One of my NBA-related Projects:
NBA AI: The Smart Assistant for Basketball Fans
NBA AI is an intelligent assistant designed for basketball enthusiasts to explore, compare, and predict NBA statistics with ease. Whether you want to check recent games, analyze team trends, compare player performances, or test your knowledge with trivia, this tool provides a seamless and interactive experience.
This project was inspired my experience in being challenged to prove my knowledge on basketball. NBA AI is built to empower fans with detailed, data-driven insights so they can confidently engage in conversations, debunk gatekeeping, and deepen their understanding of the game. With in-depth analytics, comparisons, and trivia, this AI ensures that anyone, regardless of background, can engage in meaningful conversations about the sport they love.
View my Project here.

Healthcare Oriented Projects
Data Structure Projects
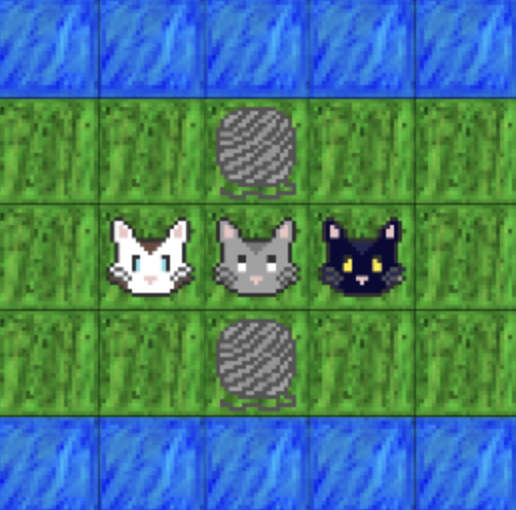
Cat Island is a Java-based simulation game made with Object-Oriented Programming (OOP). The project features a world where cats navigate islands, collecting yarn, avoiding water, and exploring different terrains. Using Java classes and objects, the program models real-world relationships between Cats, Islands, and Tiles. Each cat has movement functions, while islands are represented as 2D arrays of Tiles that track land, water, and objects. Players solve challenges by programming cat movements and modifying the environment. Key OOP principles include: Class design, encapsulation, inheritance, and polymorphism, while also incorporating recursive algorithms and game state management.
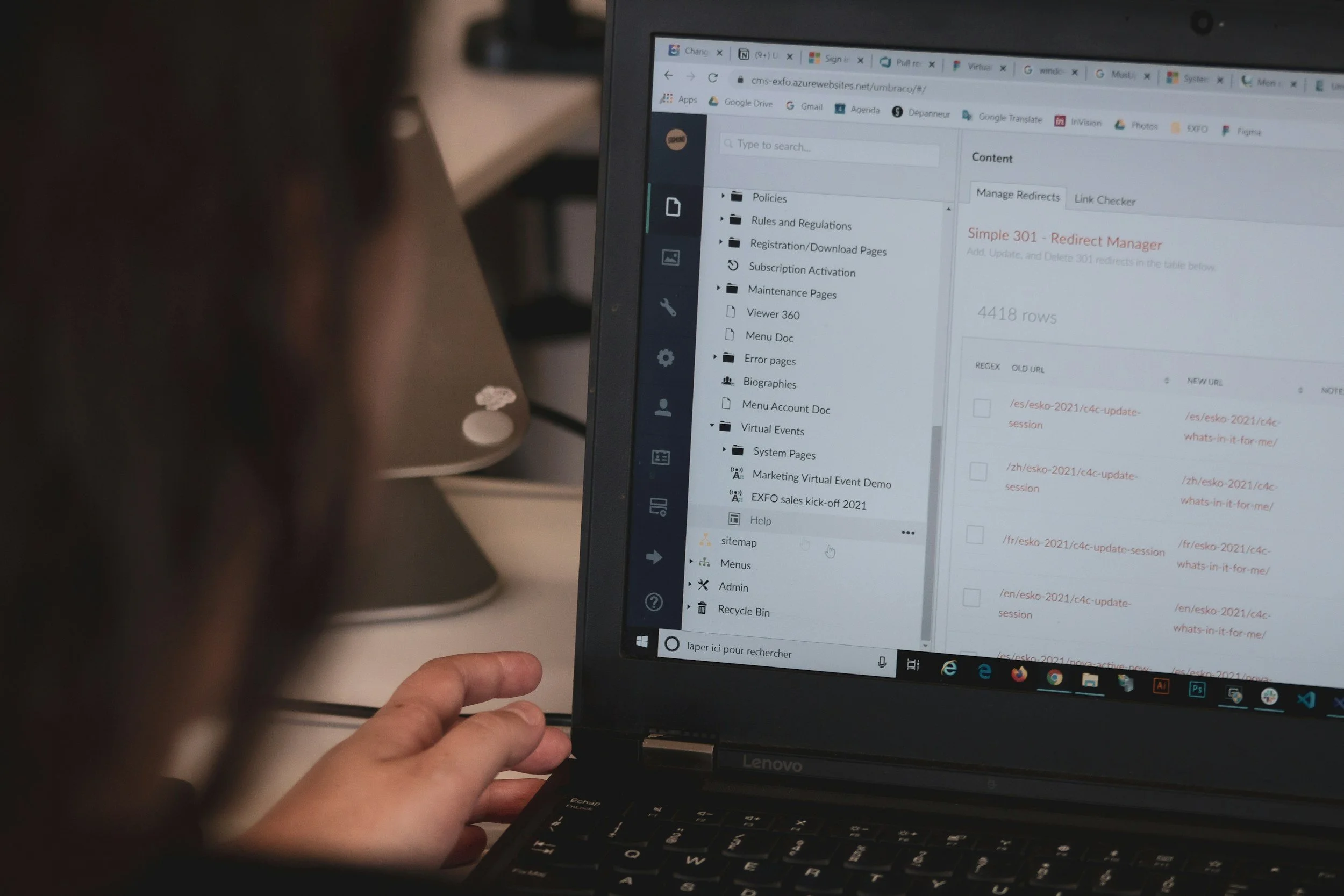
Random Cat Island is a Java-based project that showcases my debugging techniques using the Java Debugger in VS Code. This project focuses on investigating bugs by setting breakpoints, using the debug console, inspecting variables, and tracking program execution step by step. The project features a randomly generated island with cats moving across land and water, collecting yarn as the simulation progresses. Students must use debugging tools to gather information about the simulation, such as the number of land tiles, cat positions, and yarn collected at different time intervals. By exploring conditional breakpoints, the debug console, and the memory viewer, this project provides hands-on experience in troubleshooting Java programs, analyzing runtime behavior, and refining debugging skills—essential for efficient software development.

Smart Cat Island is my project to showcase mastery of JUnit testing and object-oriented inheritance in Java. I wrote JUnit test cases to verify path traversal, yarn collection, and maze-solving abilities for my CatIsland Project. The project involves creating and testing three custom islands—pathIsland, yarnIsland, and mazeIsland—using JUnit assertions to check movement, final positions, and collected yarn. This project reinforces inheritance, debugging, and test-driven development.

Theme Park Queue is a Java project focused on implementing a queue data structure using a linked list, implementing the enqueue() and dequeue() methods. The queue follows the FIFO (First-In-First-Out) principle, where new riders join at the back, and the front rider is removed when dequeued. Each rider is represented as a node in a linked list, with frontOfLine pointing to the first rider and endOfLine pointing to the last. The enqueue() method adds new riders to the end, while dequeue() removes riders from the front, updating pointers accordingly. The project includes a Driver visualization tool, allowing users to interact with the queue dynamically. I also wrote JUnit tests to verify proper queue functionality, including order preservation (FIFO), correct line length updates, and proper handling of empty queues. By implementing and testing a linked-list-based queue, this project strengthened my understanding of dynamic memory management, node-based structures, and queue operations in Java.

BST Dictionary Traversals is a Java project that focuses on implementing pre-order, post-order, in-order, and level-order traversals in a binary search tree (BST). The BST serves as a dictionary, where words act as keys and their definitions as values. Insertions follow lexicographic ordering, ensuring proper BST structure. I completed traversal methods in BSTDictionary.java, each following a distinct approach: pre-order visits nodes before their children, post-order processes children first, in-order arranges words in ascending order, and level-order uses a queue to explore nodes level by level. A Driver visualization tool allows users to interact with the BST, view its structure, and see traversal results in real-time. I wrote JUnit tests to verify correct traversal implementations by comparing expected and actual node sequences. This project reinforced key data structures concepts, including recursion, queue-based algorithms, and tree traversal techniques.
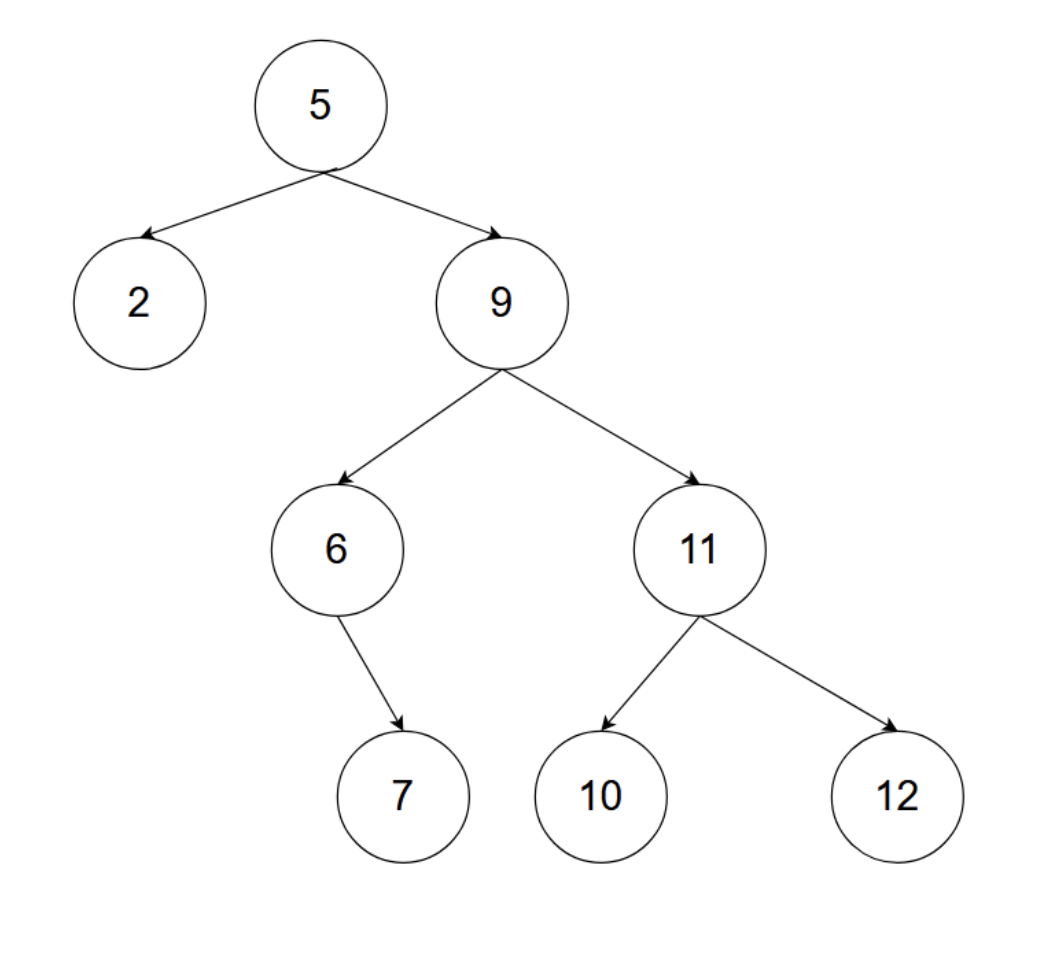
BST Dictionary Delete is a Java project focused on implementing Hibbard deletion (a method used to remove nodes from a Binary Search Tree (BST) while maintaining its structure and ordering) in a binary search tree (BST). This project builds upon previous work with BST traversals, allowing me to remove words from a dictionary stored in a BST. Each word acts as a key, following lexicographic ordering, while the deletion process ensures the BST structure remains valid. To delete a node, the program follows Hibbard deletion rules: if the node has no children, it is simply removed; if it has one child, it is replaced by that child; if it has two children, it is replaced by its inorder successor—the smallest node in its right subtree. The provided findMin() method helps locate this successor. The removeWord() method, implemented recursively, updates the tree while maintaining its ordering properties. A Driver visualization tool allowed me to add, view, and delete words, dynamically displaying changes in the BST structure. I wrote JUnit tests to validate their deletion implementation, checking edge cases such as removing leaf nodes, nodes with one child, and nodes with two children. This project reinforced recursive tree manipulation, node replacement techniques, and algorithmic problem-solving in Java.

The RU Kindergarten project is a Java-based simulation of a kindergarten classroom using linked lists, 2D arrays, and circular linked lists. I implemented and manipulated different data structures to model classroom activities such as lining up, seating arrangements, and playing musical chairs. First, I worked with a singly linked list to simulate students lining up as they enter the classroom. A 2D array represents the classroom seating, where students are placed based on available seats. The musical chairs game is implemented using a circular linked list, where students are removed one by one until a winner remains. The winner is then seated in the classroom. The project involves implementing methods to add students to the classroom, seat them, simulate musical chairs, eliminate students, and ensure proper ordering in line. A Driver visualization tool helps test and visualize student placements and game progress.
Java Projects
See descriptions of the Projects below.
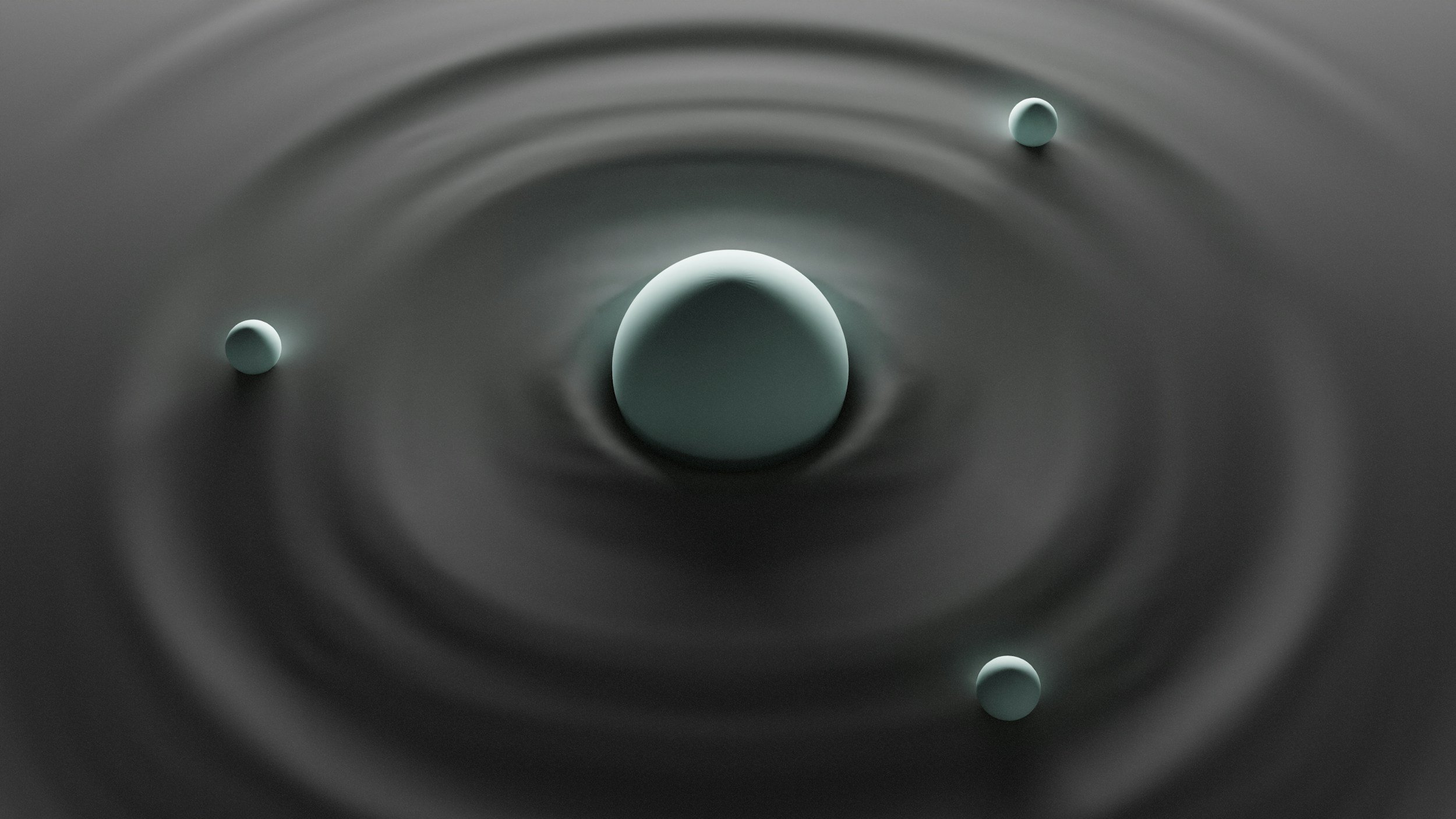
Gravitational Force: This program calculates the gravitational force between two objects using their masses and the distance between them. The program takes three double values as command-line arguments, performs the computation, and displays the result.

Palindrome: This program checks whether six integers provided as command-line arguments form a palindrome pattern. A phrase is a palindrome if it reads the same forward and backward. It uses a boolean expression to verify if the first equals the last, the second equals the second-to-last, and the third equals the third-to-last, printing true if all conditions are met and false otherwise.

Floor is Lava: This program simulates a sequence of safe spaces (stones) and dangerous spaces (lava) based on an input integer. It first prints all even numbers up to the input in ascending order (representing stones), then switches to print odd numbers in descending order (representing the reversed lava/stone state).
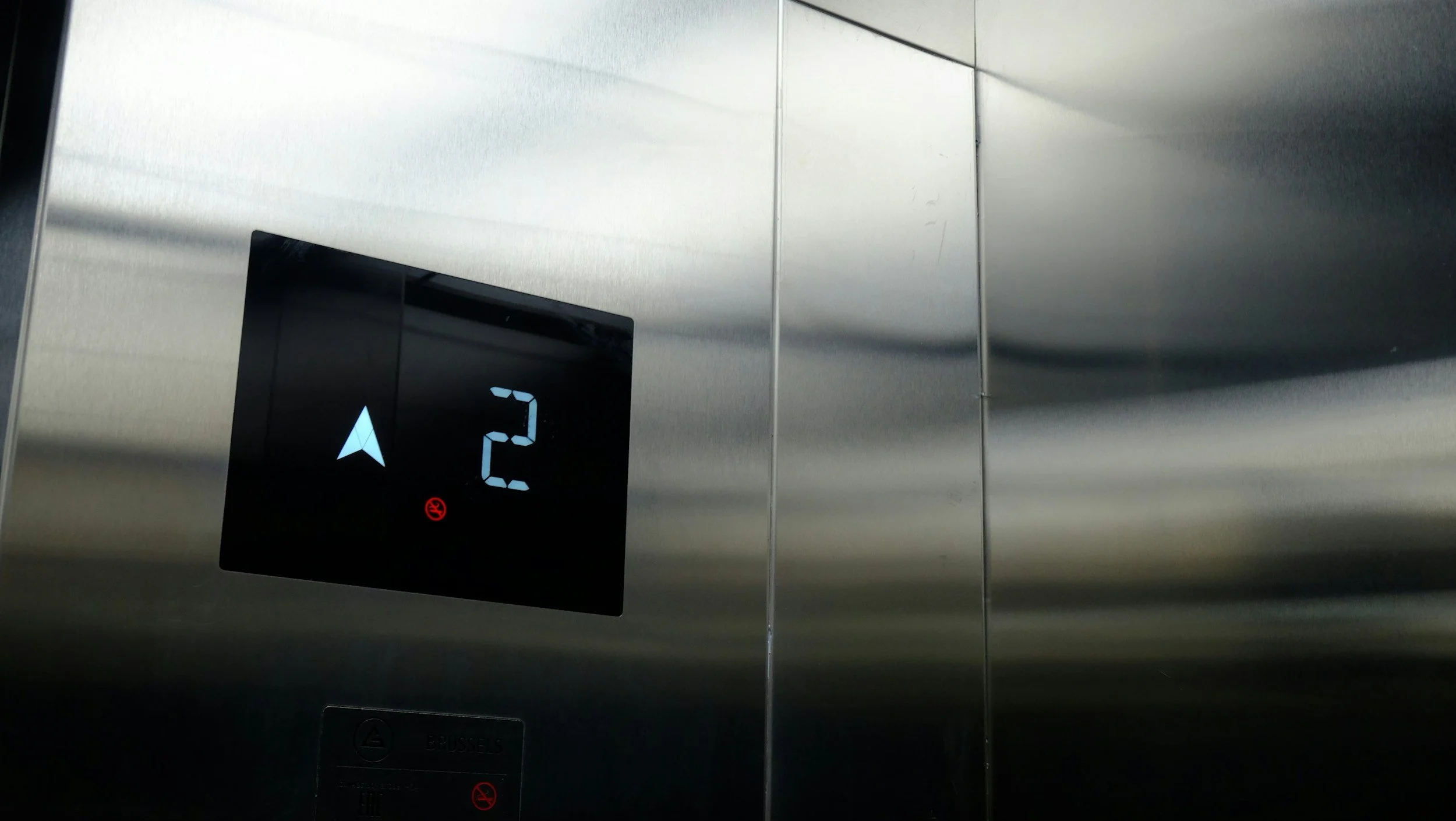
Elevator: This program simulates the operation of two elevators servicing floor requests in a building, considering proximity and priority rules. It determines which elevator services each request and handles restricted floors by validating access through an optional passcode. The program efficiently processes multiple requests, prioritizes Elevator 1 in case of ties, and outputs the elevator servicing each floor and whether access to restricted floors is granted or denied.
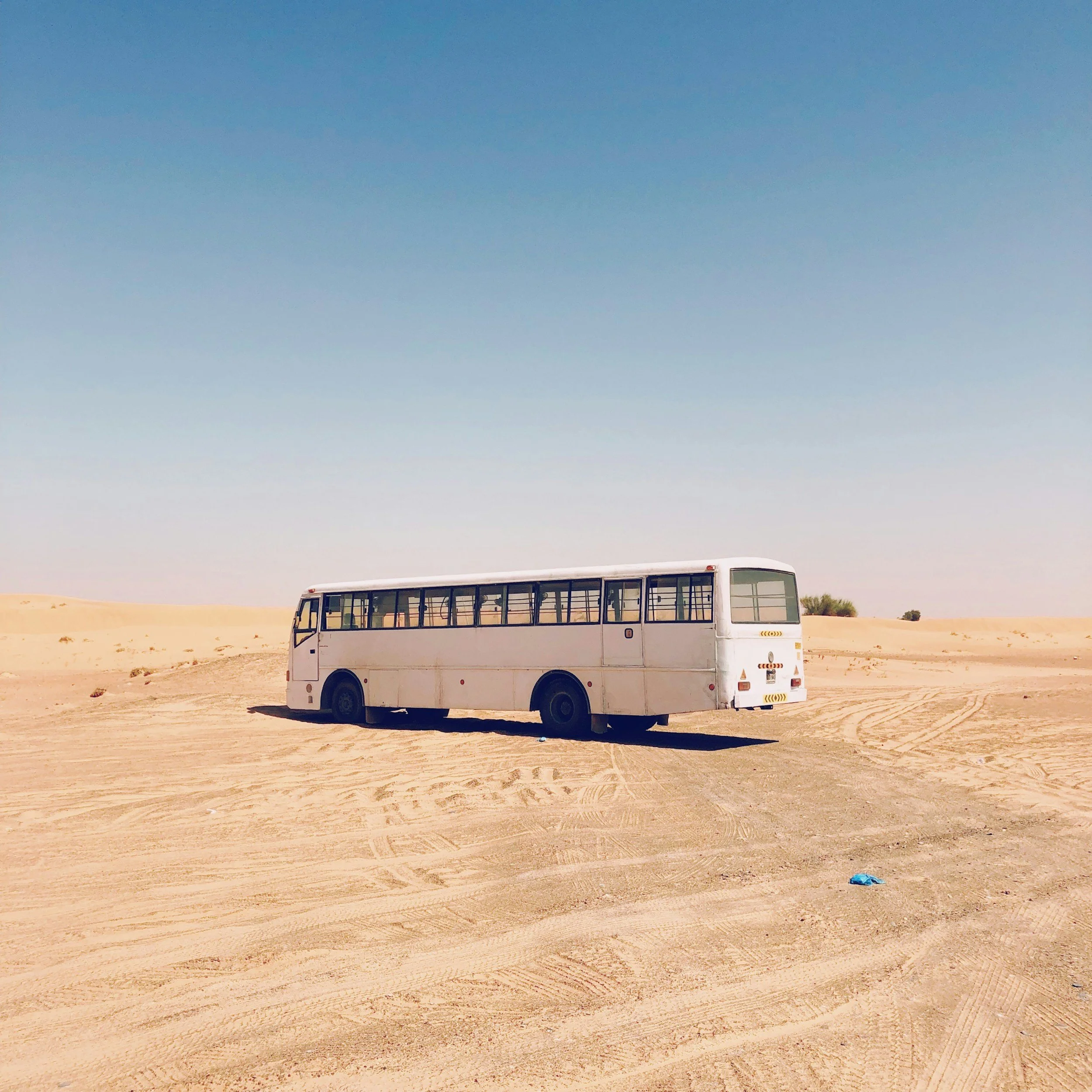
BusStop: This program determines the arrival order of buses at a stop based on a sequence of bus route names provided as input. It identifies when Serena’s desired bus will arrive or returns -1 if the bus does not stop there. The program uses a 1D array and looping techniques to search for the specified bus efficiently.

Egyptian Pyramid: This program builds a 2D pyramid grid using a specified number of bricks (X) and fills the remaining spaces with = to represent empty spots. It constructs and prints the pyramid using a 2D array, updating the grid dynamically based on the number of bricks provided. The program also outputs the number of unused bricks after the pyramid construction.

Fruit Costs: This program reads a list of fruits and their costs from an input file, identifies the two lowest-cost fruits, and calculates their total cost. Using 1D parallel arrays, the program efficiently stores and processes the fruit names and their corresponding prices.
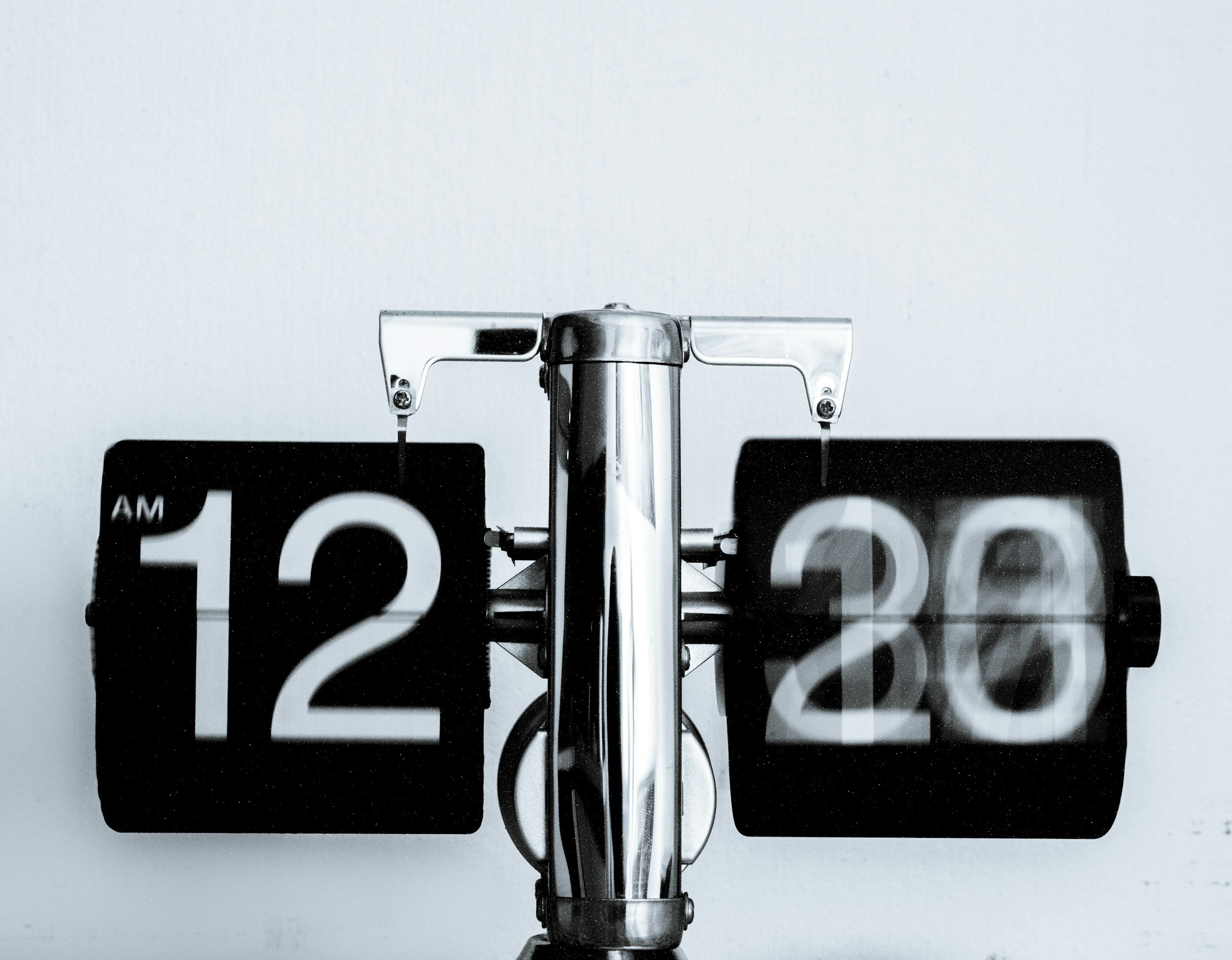
Character Counter: This program counts the occurrences of each character in a file and calculates their frequencies using a 1D integer array. The program processes ASCII characters, focusing on printable values (32–126), and writes the results to an output file in a specific format.
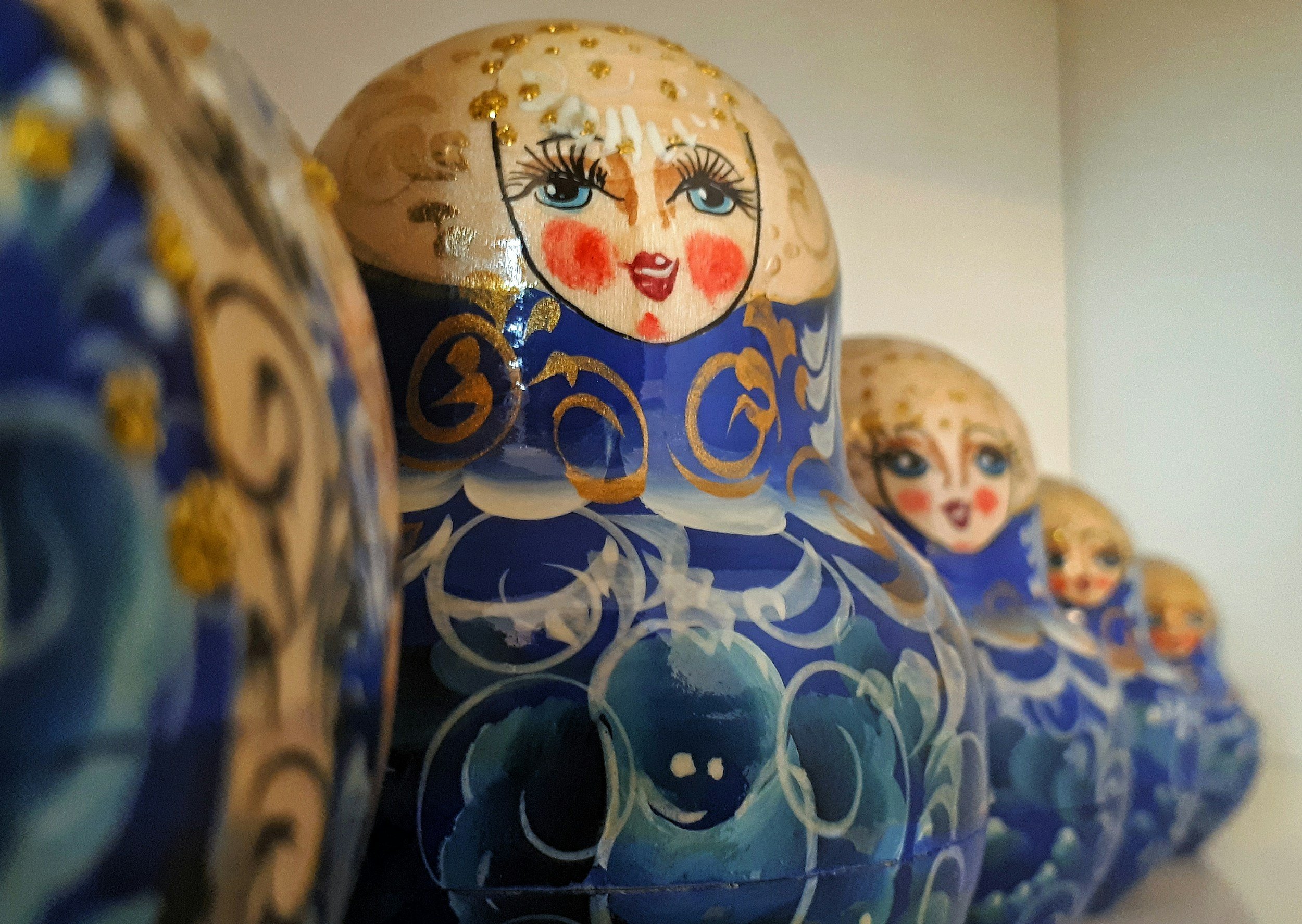
Matryoshka Doll: This program simulates the creation of Russian nesting dolls using recursion and iterative functions. It features a stackDolls() recursive method to draw a series of adjacent, progressively smaller dolls and a drawDoll() iterative method to render each doll with a head and body using StdDraw.

Quadratic Koch: This program generates a fractal pattern, the Quadratic Koch Snowflake, using recursive and iterative methods. The koch() recursive function creates segments of the snowflake, while getCoords() calculates coordinates for each segment using geometric transformations. This project focuses on recursion, fractal geometry, and 2D graphical programming.
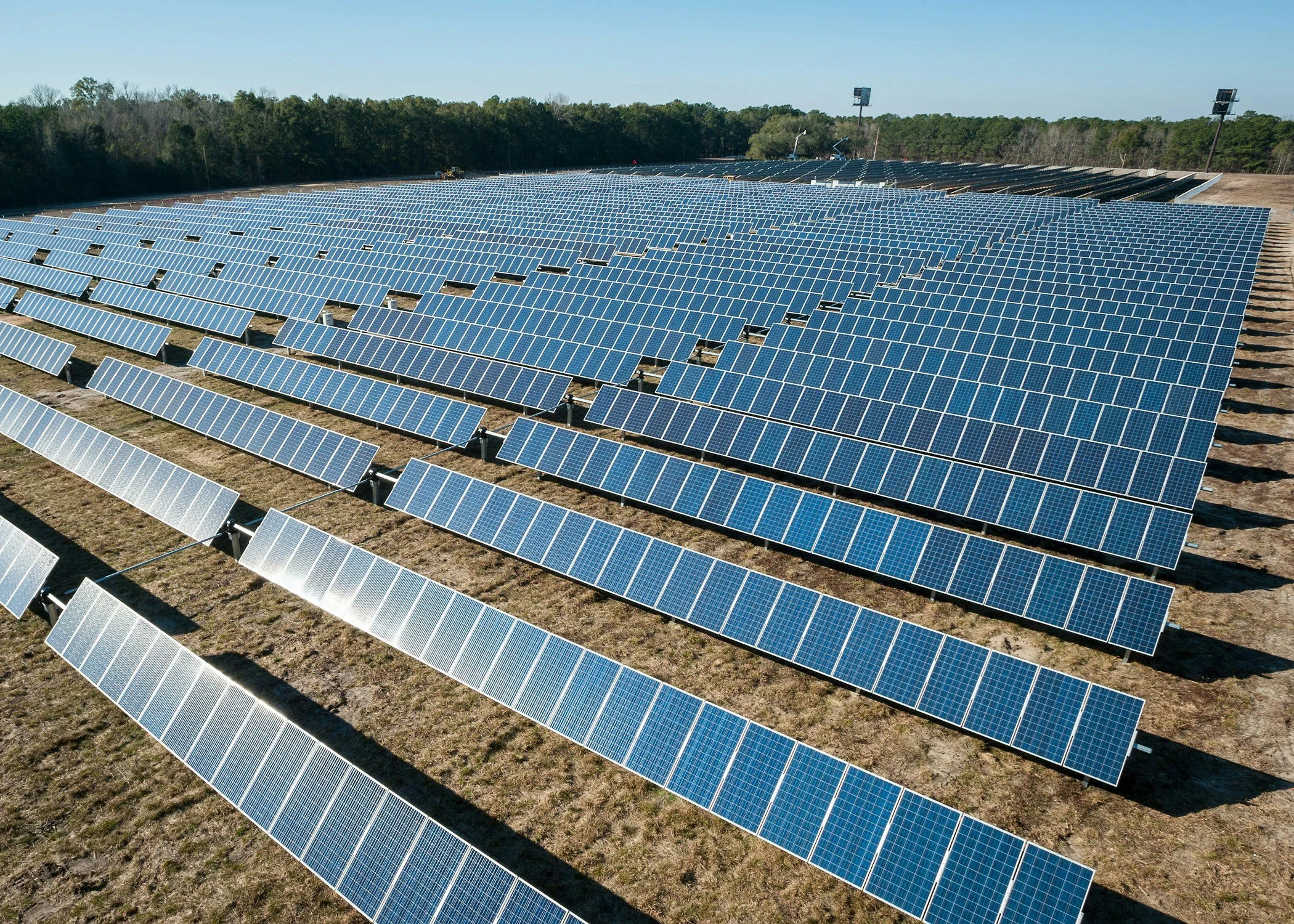
Solar Panel Consultant Project: This program simulates the process of planning, installing, and maintaining solar panel projects on Rutgers parking lots to optimize electricity generation and cost savings. It involves reading street maps and parking lot data, installing solar panels, updating their efficiency and electricity generation, and calculating Rutgers' yearly savings. This project focuses on array manipulation, 2D mapping, object-oriented programming, and energy efficiency calculations.
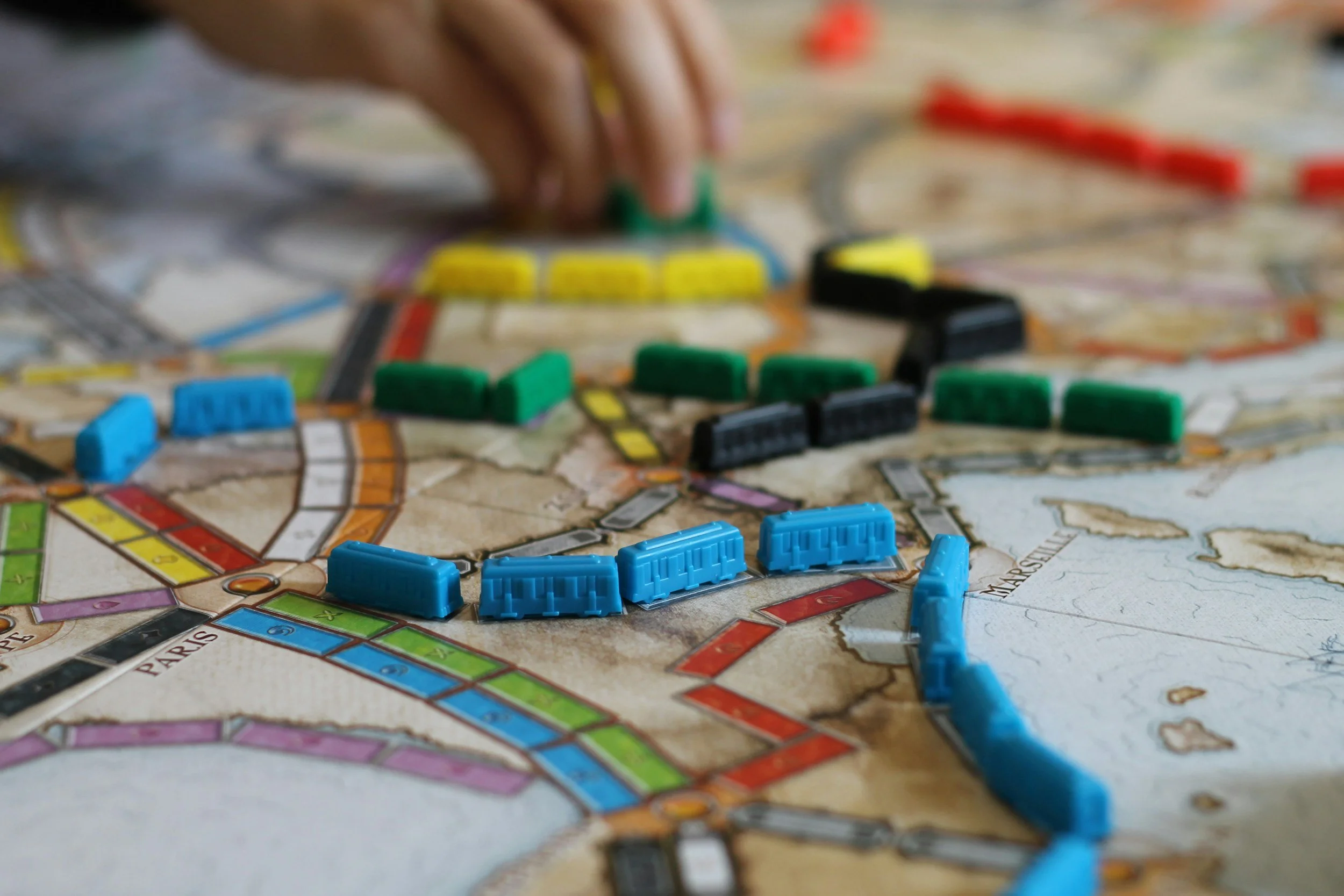
PlayMinesweeper: This program simulates the classic Minesweeper game, incorporating its rules, mechanics, and features such as mine placement, safe square identification, flagging suspected mines, and recursive opening of squares. The program uses a 2D array of Square objects to represent the game grid, and players interact through text-based or graphical interfaces to navigate the minefield.
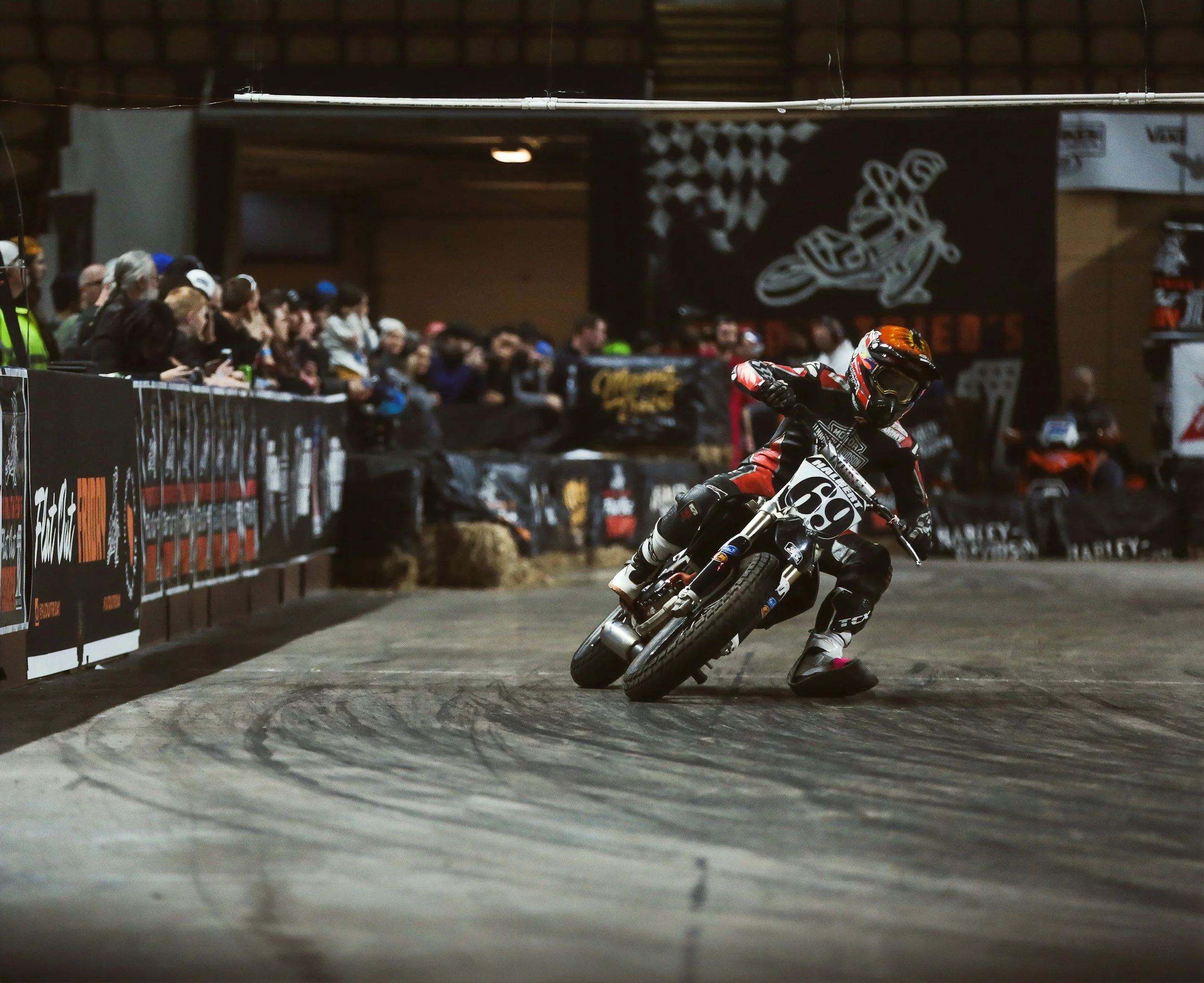
RU Racing: This program simulates racers with different time complexities (O(1), O(log n), O(n), and O(n²)) completing laps on a raceway constructed from 2D maps. Racers' behaviors illustrate algorithmic efficiencies as they navigate the raceway, demonstrating how time complexity impacts task completion.